Casio Watch with Bluetooth (nrf52832) Part 1
7/17/2023After buying a watch I noticed I began using my phone less. Eventually, I figured out it was because I wasn't using it to check the time, which would lead to checking everything else. It was like that Portlandia episode where the main guy got stuck in a loop switching between his phone and other devices. After some more thinking I noticed I only really check my phone to make sure I didn't miss a notification. With that as an excuse, I finally began working on something I've wanted to do for years: a small board with a micro controller. In this case, it would be a watch that turns on its back light when I receive a notification.
The micro controller I decided on was the Nordic nrf52832; a SoC with BLE, an impressive looking SDK, and 32 GPIO pins, which met the requirements for this project. When deciding between the various options, a friend of mine simplified the process by suggesting something from Nordic's lineup, and by pointing out that a very significant amount of the energy usage will be from the Bluetooth. Although I plan to make the design as swappable with the watch as possible, I may have to revise the design to make it rechargeable in a future project.
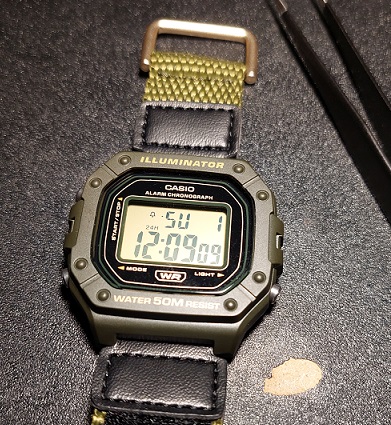
The plan is quite simple:
1. Order a dev board, a module, and a programmer
2. Figure out the firmware via the dev board
3. Make an app that forwards notifications to the chip
4. Program the result to the module
5. Design a board that is effectively the module shaped like the watch PCB, with connections to the buttons and lcd screen
This way I establish a pipeline to go from the dev board to PCB, which will simplify debugging. The industry does not typically use dev boards, but I will eat the cost this time since this will be my first time designing a PCB, or using Bluetooth in a project. I ordered the module as an intermediate just in case. The programmer is an STLink V2 knock-off, which I chose because of the price.
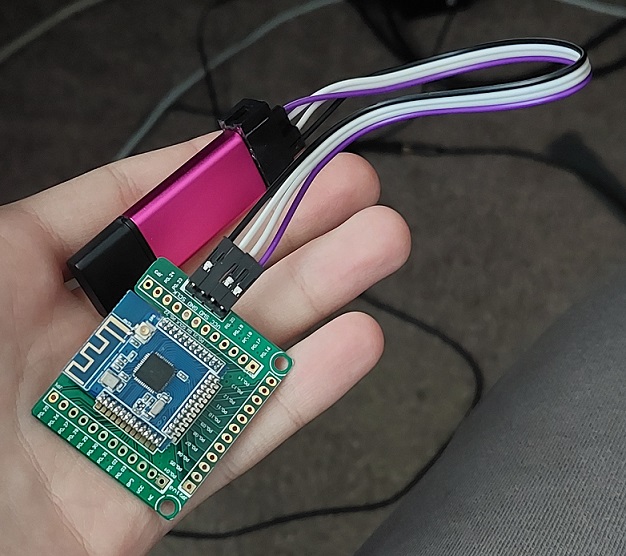
Once the boards arrived I was faced with the decision of which IDE to use, and while I initially thought it may require some windows proprietary IDE (Atmel Studio?) I settled on VSCode when I learned about the nRF Connect extension. In retrospect, the tool I used for flashing the module is also a Linux tool (openocd), so I could have done this project on Linux. I did learn that it isn't that different than Windows anyhow, at least at the level I'm at.
Reading material about BLE from Nordic, Bluetooth Low Energy: The Developer's Handbook (Robin Heydon, 2012), and online had made me initially think there would be a standard BLE service for my use-case. What further mislead me was the Alert Notification Service, which at first glance seemed like it would be perfect. In actuality, this is a server service which can be used to send notifications, rather than a client service that would listen to notifications. After realizing this, I finally decided I would need to make an app to do the forwarding.
The dev board is an incredible resource, and there are many example projects available for it, along with an app you can use to test the firmware you write. Working from the peripheral_lbs example I was able to write a custom service to control an LED based on a characteristic with write permissions, and functionality that would clear the LED whenever a button is pressed. Thankfully, this also worked an the module after I programmed the elf file to it that was generated by nRF Connect, after modifying the program to use a GPIO pin for the LED signal.
Writing the app was a bit more of a hassle, and it is still very bare bones. The most confusing bug was that even after the notification listener service was initialized, the notifications weren't triggering the relevant callback. Thankfully, I found a stack overflow thread that suggested adding a pause right after initializing the service and before assigning the callback, which fixed the issue. This is very hacky and I'm sure there is a better way, but it's a proof of concept, and will likely need to be re-written anyways. Perhaps with a framework to handle bluetooth edge cases like the one just mentioned, and it would preferably be written in Flutter for interoperability with IOS. As it stands, this has been a good opportunity to dip my feet into Kotlin, that which I agree with the general sentiment is far superior to Java.
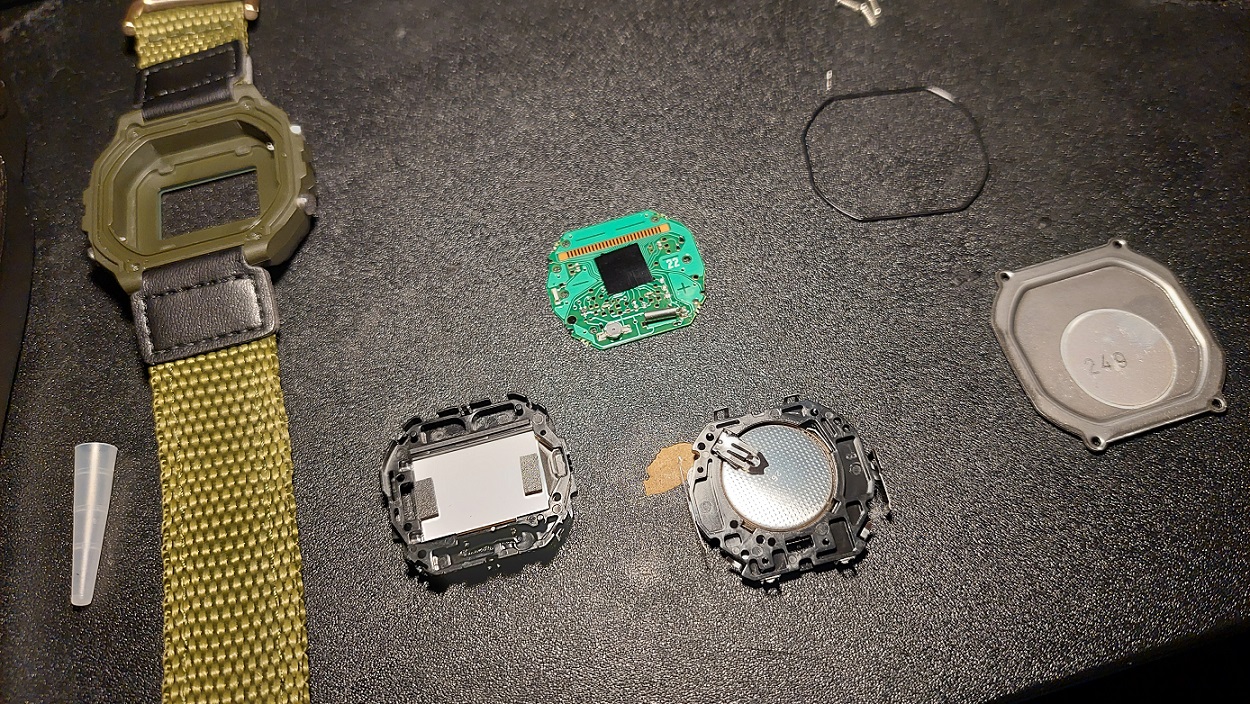
Designing the PCB was fun, sort of like a puzzle because of how small it was. I used the Easy EDA editor because it seemed rather beginner friendly and integrated with the JLC marketplace, so it was easier to order the parts afterwards. The design I used was just a copy of the reference schematic provided by Nordic, with a STLink interface and the GPIO pins routed to the proper pads.
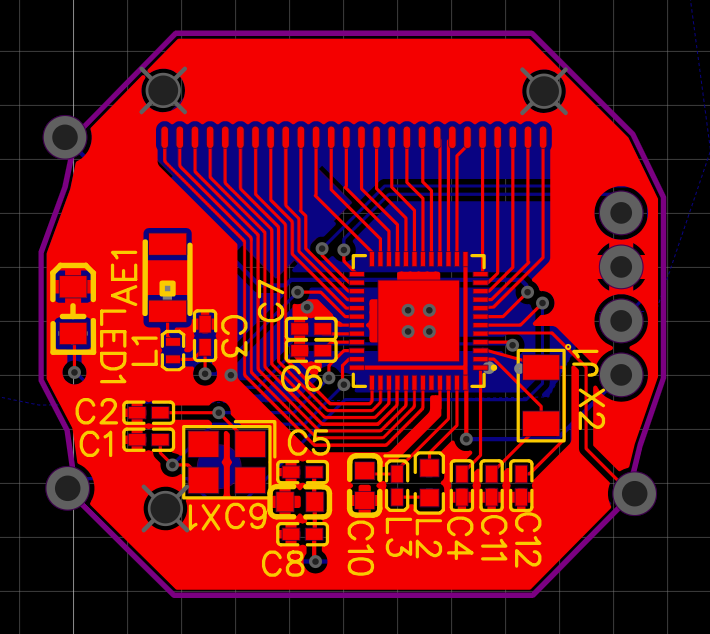
The most tricky part was getting the measurements as accurate as possible to the watch PCB because, frankly, the PCB designer built into Easy EDA in not designed for making accurate shapes. I found I could sketch the shape in Fusion 360 and import it as a DXF file, which was a much more intuitive experience. In retrospect I could have used Fusion 360 for the whole design, but I had already made the whole schematic in Easy EDA. I guess next time. I verified the design by printing it on paper and holding it to the watch PCB; it's not high tech but it does the job.
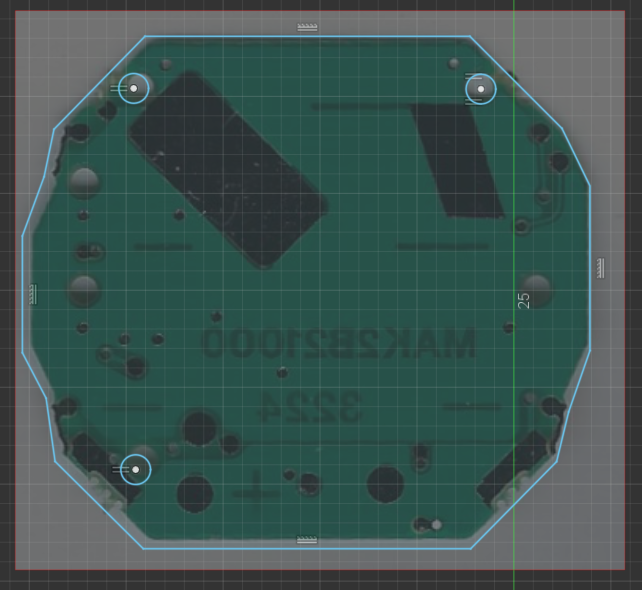
The watch handles buttons by having plated edges at 3 corners of the board that come in contact with metal folds when the buttons are pressed. I replicated this by adding through holes at those places, at the very edge. I got an email after I placed my order for the PCB asking if I would like to have those edges plated at an additional cost of around $50 for my $3 order, which I declined. If they aren't plated this time around I'll extend the edge a bit and cut through the through holes.
While I wait for the PCBs to come in I don't have much to do, so I tried experimenting further on the module. Sadly, I did destroy it when I ran 5 volts through a GPIO pin, which is very much past the absolute limits on the nrf52832's data sheet. What's important is the module proved my earlier theories anyhow, I'm confident if there aren't any issues with the PCB I will be able to program it with the same method I did the module. Examining it showed it was very much just the reference design, anyways.